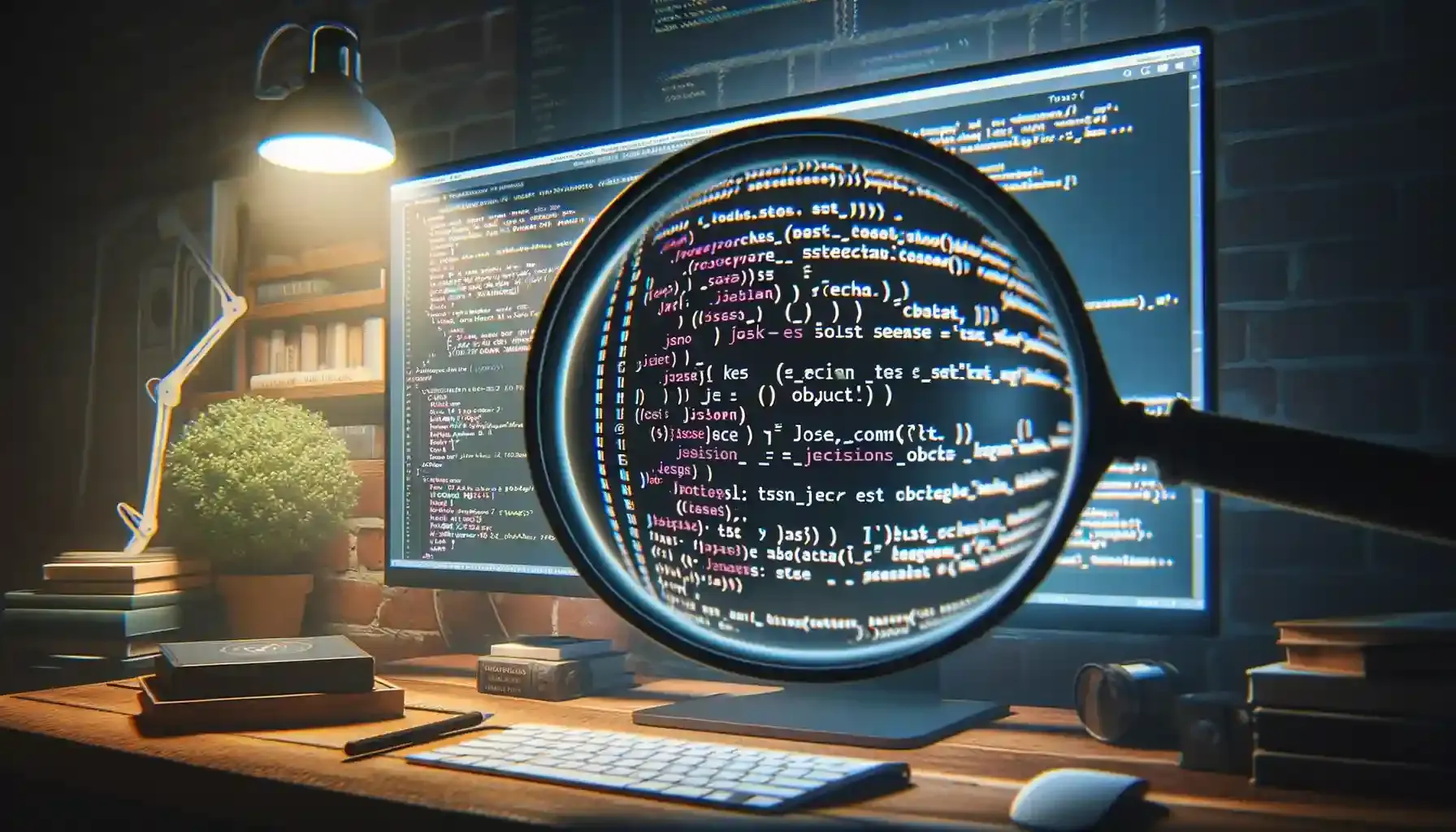
How to find a specific object key in jest
Sep 03 2022
//Updated Oct 29 2023
//2 min read
We’ve all been there, writing a jest test and having that one case where we want to have a very specific key in an object.
our first attempt might be
it("should redirect to correct url if id is incorrect", () => {
const mockFn = jest.fn()
mockRouter.query = {
id: "id2",
}
mockRouter.push = mockFn
render(<Component>I am visible</Component>)
expect(mockFn).toHaveBeenCalledWith({
query: { id: "id1" },
})
})
This will throw us an error like this
Error: expect(jest.fn()) .toHaveBeenCalledWith(...expected)
Solution
Since Jest 18 we are able to use objectContaining . This makes it super easy to check for 1 or more specific keys:
it("should redirect to correct url if id is incorrect", () => {
const mockFn = jest.fn()
mockRouter.query = {
id: "id2",
}
mockRouter.push = mockFn
render(<Component>I am visible</Component>)
expect(mockFn).toHaveBeenCalledWith(
expect.objectContaining({
query: { id: "id1" },
})
)
})
this test will pass.
Alternative solutions
in the case that you only have a few keys you could also use expect.anything()
this would be used like so:
expect(mockFn).toHaveBeenCalledWith({
query: { id: "id1" },
key: expect.anything(),
key2: expect.anything()
})
Conclusion
there are 2 options to find a specific key in objects. objectContaining and expect.anything()